Software Development
Showing 97–108 of 2824 results
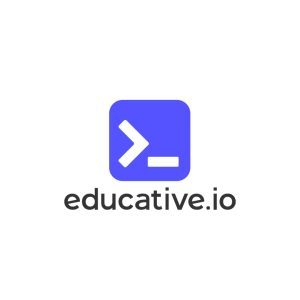
Angular: Designing and Architecting Web Applications
Learn how to develop Angular applications with TypeScript. Understand concepts such as components, pipes, directives, observables.
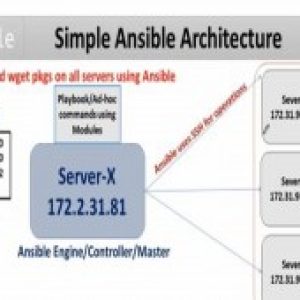
Ansible & Ansible-Playbooks For Automation
Ansible to automate local and cloud configuration management tasks with Playbooks

Ansible 2 and DevOps Integrations: The Big Picture
Companies realize that automation leads to acceleration. This course will teach you to gain a big picture of the different tools and technologies supported by Ansible in automating a DevOps pipeline.
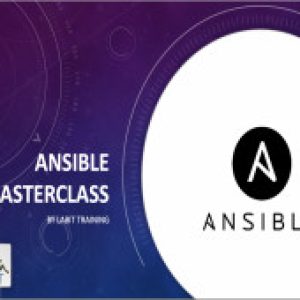
Ansible for an Absolute Beginner – Automation – DevOps
Learn Ansible from scratch as an absolute beginner in DevOps using hands-on practice exercises
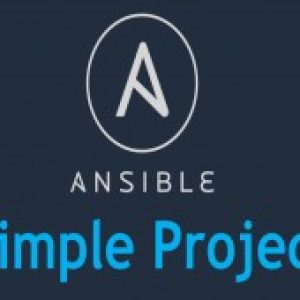
Ansible for the DevOps Beginners & System Admins
Ansible for the Beginners course for DevOps Engineers & System Admins helps you to enhance skills towards Ansible.

Ansible on Windows Fundamentals
This course equips you with the tools to automate and manage Windows infrastructure seamlessly. This course guides you through creating Ansible playbooks, leveraging roles, and harnessing advanced connection configurations for optimal results.

Anti-aging: Keeping Your Code Young and Thriving
This session focuses on slow code quality decreases over time, why it happens, and how you can combat it.
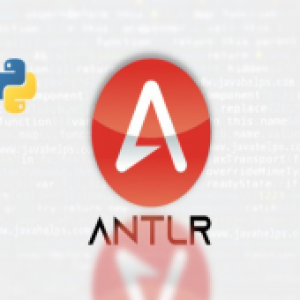
ANTLR Programming Masterclass with Python
ANTLR Programming Masterclass with Python

Apache API Integration in Java Fundamentals
PDFBox and POI are Apache’s leading open source Java APIs for working with PDF documents and Microsoft documents. This course will teach you how to use Apache APIs with PDFs and Microsoft documents in a Java application of any scale.

Apex Academy: Absolute Beginner’s Guide to Coding in Salesforce
Anyone can code in Salesforce! A crash course on how to code Apex and why learning to code will skyrocket your career (and wallet)!

Apex Academy: Bulkification and Governor Limits
Salesforce requires precisely executed design best practices to avoid colliding with governor limits, which will bring Salesforce transactions to a halt. This course will teach you how to avoid colliding with governor limits while developing new object transactions or troubleshooting existing designs.

Apex Academy: Fundamental Salesforce Coding Techniques
Learn the core coding concepts that you'll be using every day in Salesforce! Practice using core development tools such as variables, IF statements, loops, and more.